|
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778798081828384858687888990919293949596979899100101102103104105106107108109110111112113114115116117118119120121122123124125126127128129130131132133134135136137138139140141142143144145146147148149150151152153154155156157158159160161162163164165166167168169170171172173174175176177178179180181182183184185186187188189190191192193194195196197198199200201202203204205206207208209210211212213214215216217218219220221222223224225226227228229230231232233234235236237238239240241242243244245246247248249250251252253254255256257258259260261262263264265266267268269270271272273274 |
- # ansi-colors [](https://www.npmjs.com/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://travis-ci.org/doowb/ansi-colors) [](https://ci.appveyor.com/project/doowb/ansi-colors)
-
- > Easily add ANSI colors to your text and symbols in the terminal. A faster drop-in replacement for chalk, kleur and turbocolor (without the dependencies and rendering bugs).
-
- Please consider following this project's author, [Brian Woodward](https://github.com/doowb), and consider starring the project to show your :heart: and support.
-
- ## Install
-
- Install with [npm](https://www.npmjs.com/):
-
- ```sh
- $ npm install --save ansi-colors
- ```
-
- 
-
- ## Why use this?
-
- ansi-colors is _the fastest Node.js library for terminal styling_. A more performant drop-in replacement for chalk, with no dependencies.
-
- * _Blazing fast_ - Fastest terminal styling library in node.js, 10-20x faster than chalk!
-
- * _Drop-in replacement_ for [chalk](https://github.com/chalk/chalk).
- * _No dependencies_ (Chalk has 7 dependencies in its tree!)
-
- * _Safe_ - Does not modify the `String.prototype` like [colors](https://github.com/Marak/colors.js).
- * Supports [nested colors](#nested-colors), **and does not have the [nested styling bug](#nested-styling-bug) that is present in [colorette](https://github.com/jorgebucaran/colorette), [chalk](https://github.com/chalk/chalk), and [kleur](https://github.com/lukeed/kleur)**.
- * Supports [chained colors](#chained-colors).
- * [Toggle color support](#toggle-color-support) on or off.
-
- ## Usage
-
- ```js
- const c = require('ansi-colors');
-
- console.log(c.red('This is a red string!'));
- console.log(c.green('This is a red string!'));
- console.log(c.cyan('This is a cyan string!'));
- console.log(c.yellow('This is a yellow string!'));
- ```
-
- 
-
- ## Chained colors
-
- ```js
- console.log(c.bold.red('this is a bold red message'));
- console.log(c.bold.yellow.italic('this is a bold yellow italicized message'));
- console.log(c.green.bold.underline('this is a bold green underlined message'));
- ```
-
- 
-
- ## Nested colors
-
- ```js
- console.log(c.yellow(`foo ${c.red.bold('red')} bar ${c.cyan('cyan')} baz`));
- ```
-
- 
-
- ### Nested styling bug
-
- `ansi-colors` does not have the nested styling bug found in [colorette](https://github.com/jorgebucaran/colorette), [chalk](https://github.com/chalk/chalk), and [kleur](https://github.com/lukeed/kleur).
-
- ```js
- const { bold, red } = require('ansi-styles');
- console.log(bold(`foo ${red.dim('bar')} baz`));
-
- const colorette = require('colorette');
- console.log(colorette.bold(`foo ${colorette.red(colorette.dim('bar'))} baz`));
-
- const kleur = require('kleur');
- console.log(kleur.bold(`foo ${kleur.red.dim('bar')} baz`));
-
- const chalk = require('chalk');
- console.log(chalk.bold(`foo ${chalk.red.dim('bar')} baz`));
- ```
-
- **Results in the following**
-
- (sans icons and labels)
-
- 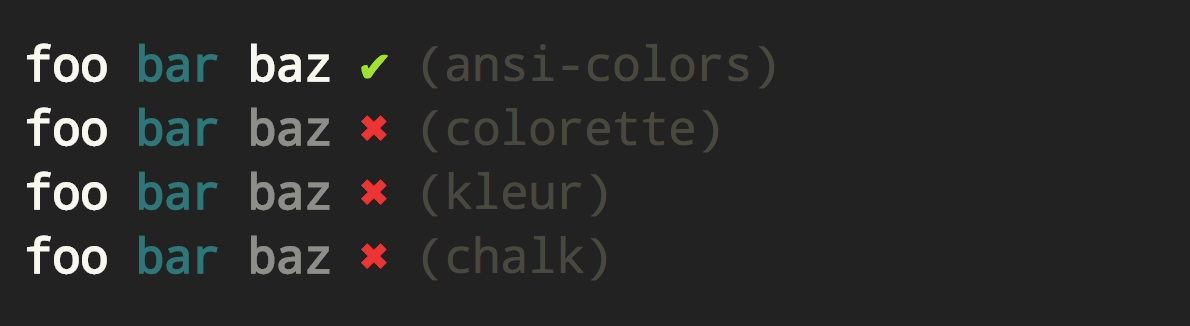
-
- ## Toggle color support
-
- Easily enable/disable colors.
-
- ```js
- const c = require('ansi-colors');
-
- // disable colors manually
- c.enabled = false;
-
- // or use a library to automatically detect support
- c.enabled = require('color-support').hasBasic;
-
- console.log(c.red('I will only be colored red if the terminal supports colors'));
- ```
-
- ## Strip ANSI codes
-
- Use the `.unstyle` method to strip ANSI codes from a string.
-
- ```js
- console.log(c.unstyle(c.blue.bold('foo bar baz')));
- //=> 'foo bar baz'
- ```
-
- ## Available styles
-
- **Note** that bright and bright-background colors are not always supported.
-
- | Colors | Background Colors | Bright Colors | Bright Background Colors |
- | ------- | ----------------- | ------------- | ------------------------ |
- | black | bgBlack | blackBright | bgBlackBright |
- | red | bgRed | redBright | bgRedBright |
- | green | bgGreen | greenBright | bgGreenBright |
- | yellow | bgYellow | yellowBright | bgYellowBright |
- | blue | bgBlue | blueBright | bgBlueBright |
- | magenta | bgMagenta | magentaBright | bgMagentaBright |
- | cyan | bgCyan | cyanBright | bgCyanBright |
- | white | bgWhite | whiteBright | bgWhiteBright |
- | gray | | | |
- | grey | | | |
-
- _(`gray` is the U.S. spelling, `grey` is more commonly used in the Canada and U.K.)_
-
- ### Style modifiers
-
- * dim
- * **bold**
-
- * hidden
- * _italic_
-
- * underline
- * inverse
- * ~~strikethrough~~
-
- * reset
-
- ## Performance
-
- **Libraries tested**
-
- * ansi-colors v3.0.4
- * chalk v2.4.1
-
- ### Mac
-
- > MacBook Pro, Intel Core i7, 2.3 GHz, 16 GB.
-
- **Load time**
-
- Time it takes to load the first time `require()` is called:
-
- * ansi-colors - `1.915ms`
- * chalk - `12.437ms`
-
- **Benchmarks**
-
- ```
- # All Colors
- ansi-colors x 173,851 ops/sec ±0.42% (91 runs sampled)
- chalk x 9,944 ops/sec ±2.53% (81 runs sampled)))
-
- # Chained colors
- ansi-colors x 20,791 ops/sec ±0.60% (88 runs sampled)
- chalk x 2,111 ops/sec ±2.34% (83 runs sampled)
-
- # Nested colors
- ansi-colors x 59,304 ops/sec ±0.98% (92 runs sampled)
- chalk x 4,590 ops/sec ±2.08% (82 runs sampled)
- ```
-
- ### Windows
-
- > Windows 10, Intel Core i7-7700k CPU @ 4.2 GHz, 32 GB
-
- **Load time**
-
- Time it takes to load the first time `require()` is called:
-
- * ansi-colors - `1.494ms`
- * chalk - `11.523ms`
-
- **Benchmarks**
-
- ```
- # All Colors
- ansi-colors x 193,088 ops/sec ±0.51% (95 runs sampled))
- chalk x 9,612 ops/sec ±3.31% (77 runs sampled)))
-
- # Chained colors
- ansi-colors x 26,093 ops/sec ±1.13% (94 runs sampled)
- chalk x 2,267 ops/sec ±2.88% (80 runs sampled))
-
- # Nested colors
- ansi-colors x 67,747 ops/sec ±0.49% (93 runs sampled)
- chalk x 4,446 ops/sec ±3.01% (82 runs sampled))
- ```
-
- ## About
-
- <details>
- <summary><strong>Contributing</strong></summary>
-
- Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
-
- </details>
-
- <details>
- <summary><strong>Running Tests</strong></summary>
-
- Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
-
- ```sh
- $ npm install && npm test
- ```
-
- </details>
-
- <details>
- <summary><strong>Building docs</strong></summary>
-
- _(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
-
- To generate the readme, run the following command:
-
- ```sh
- $ npm install -g verbose/verb#dev verb-generate-readme && verb
- ```
-
- </details>
-
- ### Related projects
-
- You might also be interested in these projects:
-
- * [ansi-wrap](https://www.npmjs.com/package/ansi-wrap): Create ansi colors by passing the open and close codes. | [homepage](https://github.com/jonschlinkert/ansi-wrap "Create ansi colors by passing the open and close codes.")
- * [strip-color](https://www.npmjs.com/package/strip-color): Strip ANSI color codes from a string. No dependencies. | [homepage](https://github.com/jonschlinkert/strip-color "Strip ANSI color codes from a string. No dependencies.")
-
- ### Contributors
-
- | **Commits** | **Contributor** |
- | --- | --- |
- | 38 | [jonschlinkert](https://github.com/jonschlinkert) |
- | 38 | [doowb](https://github.com/doowb) |
- | 6 | [lukeed](https://github.com/lukeed) |
- | 2 | [Silic0nS0ldier](https://github.com/Silic0nS0ldier) |
- | 1 | [dwieeb](https://github.com/dwieeb) |
- | 1 | [jorgebucaran](https://github.com/jorgebucaran) |
- | 1 | [madhavarshney](https://github.com/madhavarshney) |
- | 1 | [Weishi93](https://github.com/Weishi93) |
- | 1 | [chapterjason](https://github.com/chapterjason) |
-
- ### Author
-
- **Brian Woodward**
-
- * [GitHub Profile](https://github.com/doowb)
- * [Twitter Profile](https://twitter.com/doowb)
- * [LinkedIn Profile](https://linkedin.com/in/woodwardbrian)
-
- ### License
-
- Copyright © 2018, [Brian Woodward](https://github.com/doowb).
- Released under the [MIT License](LICENSE).
-
- ***
-
- _This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.8.0, on December 03, 2018._
|